Java Math
Java Tutorial » Java Math
Java Math class contains methods to perform basic numeric operations as: elementary exponential, square root, logarithm, and trigonometric functions.
Java supply several methods to work with math calculations as: min(), max(), avg(), cos() ,sin() tan(), ceil(), round(), abs(), floor() etc.
Java Math class, example
Example:
a = 36,
b = 9,
1. Highest number between a and b is: 36.0
2. Square root of a is: 6.0
3. Power of a and b is: 1.01559956668416E14
4. Logarithm of a is: 3.58351893845611
4.1 Logarithm of b is: 2.1972245773362196
5. exp of a is: 4.311231547115195E15
6. Random number between 0 and 998 is: 728
public class Main {
public static void main(String[] args) {
double a = 36;
double b = 9;
System.out.println("a = 36,");
System.out.println("b = 9,");
// max(a,b) method - find the highest value of a and b:
System.out.println("1. Highest number between a and b is: " +Math.max(a, b));
// return the square root of a
System.out.println("2. Square root of a is: " + Math.sqrt(a));
//returns 36 power of 9 = 36*36*36*36*36*36*36*36*36
System.out.println("3. Power of a and b is: " + Math.pow(a, b));
// return the logarithm of given value
System.out.println("4. Logarithm of a is: " + Math.log(a));
System.out.println("4.1 Logarithm of b is: " + Math.log(b));
// return a power of 2
System.out.println("5. exp of a is: " +Math.exp(a));
// random number between 0 and 998
int randomNum = (int)(Math.random() * 998);
System.out.println("6. Random number between 0 and 998 is: " +randomNum);
}
}
Output:public static void main(String[] args) {
double a = 36;
double b = 9;
System.out.println("a = 36,");
System.out.println("b = 9,");
// max(a,b) method - find the highest value of a and b:
System.out.println("1. Highest number between a and b is: " +Math.max(a, b));
// return the square root of a
System.out.println("2. Square root of a is: " + Math.sqrt(a));
//returns 36 power of 9 = 36*36*36*36*36*36*36*36*36
System.out.println("3. Power of a and b is: " + Math.pow(a, b));
// return the logarithm of given value
System.out.println("4. Logarithm of a is: " + Math.log(a));
System.out.println("4.1 Logarithm of b is: " + Math.log(b));
// return a power of 2
System.out.println("5. exp of a is: " +Math.exp(a));
// random number between 0 and 998
int randomNum = (int)(Math.random() * 998);
System.out.println("6. Random number between 0 and 998 is: " +randomNum);
}
}
a = 36,
b = 9,
1. Highest number between a and b is: 36.0
2. Square root of a is: 6.0
3. Power of a and b is: 1.01559956668416E14
4. Logarithm of a is: 3.58351893845611
4.1 Logarithm of b is: 2.1972245773362196
5. exp of a is: 4.311231547115195E15
6. Random number between 0 and 998 is: 728
Java Math random, library, pow, round up, abs, function, floor, sqrt, round, ceil
Java Math - java
Online Editor
This tool makes it easy to create, adjust, and experiment with custom colors for the web.
HTML Templates

Magnews2 is a modern and creative free magazine and news website template that will help you kick off your online project in style.
CSS HTML Layout
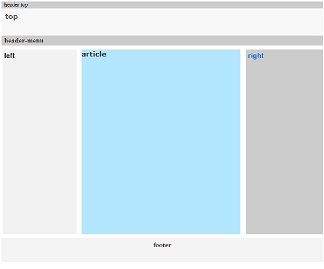
Find here examples of creative and unique website layouts.
Free CSS HTML Menu
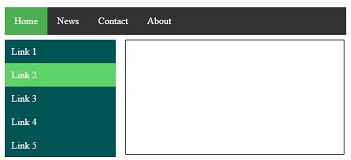
Find here examples of creative and unique website CSS HTML menu.