Java For Loop
Java Tutorial » Java For Loop
for loop is used to run a block of code for a certain number of times.
For loop is used when you know the exact number of code repetitions.
Syntax:
for (initialExpression; testExpression; updateExpression) {
// code block to be executed
}
// code block to be executed
}
initialExpression is executed (one time) before the execution of the code block.
testExpression defines the condition for executing the code block.
updateExpression is executed (every time) after the code block has been executed.
Example: will print the numbers 1 to 5
2
3
4
5
public class Main {
public static void main(String[] args) {
for (int x = 1; x < 6; x++) {
System.out.println(x);
}
}
}
1public static void main(String[] args) {
for (int x = 1; x < 6; x++) {
System.out.println(x);
}
}
}
2
3
4
5
Java For-Each Loop, example
"for-each" loop, is used exclusively to loop through elements in an array:
Syntax: Do / While Loop.
for(Type varName : arrayname){
//code or block to be executed
}
//code or block to be executed
}
example outputs all elements in the color array, using "for-each" loop:
Example: for-each Loop.
red
blue
black
white
public class Main {
public static void main(String[] args) {
String[] colors = {"red", "blue", "black", "white"};
for (String i : colors) {
System.out.println(i);
}
}
}
Output: public static void main(String[] args) {
String[] colors = {"red", "blue", "black", "white"};
for (String i : colors) {
System.out.println(i);
}
}
}
red
blue
black
white
Java Nested For Loop
If we have "for" loop inside to another loop, it is known as nested for loop.
Example: Nested For Loop Pyramid 1.
*
* *
* * *
* * * *
* * * * *
* * * * * *
public class Main {
public static void main(String[] args) {
for(int x=1; x<=5; x++){
for(int y=1; y<=i; y++){
System.out.print("* ");
}
System.out.println();//new line
}
}
}
Output: public static void main(String[] args) {
for(int x=1; x<=5; x++){
for(int y=1; y<=i; y++){
System.out.print("* ");
}
System.out.println();//new line
}
}
}
*
* *
* * *
* * * *
* * * * *
* * * * * *
Nested For Loop Pyramid 2.
Example: Nested For Loop Pyramid 2.
* * * * * *
* * * * *
* * * *
* * *
* *
*
public class Main {
public static void main(String[] args) {
int term=6;
for(int x=1;x<=term;x++){
for(int y=term;y>=x;y--){
System.out.print("* ");
}
System.out.println();//new line
}
}
}
Output: public static void main(String[] args) {
int term=6;
for(int x=1;x<=term;x++){
for(int y=term;y>=x;y--){
System.out.print("* ");
}
System.out.println();//new line
}
}
}
* * * * * *
* * * * *
* * * *
* * *
* *
*
Java For Loop array, arraylist, example, break, list, exit, types, variants, iterator, for string
Java For Loop - java
Online Editor
This tool makes it easy to create, adjust, and experiment with custom colors for the web.
HTML Templates

Magnews2 is a modern and creative free magazine and news website template that will help you kick off your online project in style.
CSS HTML Layout
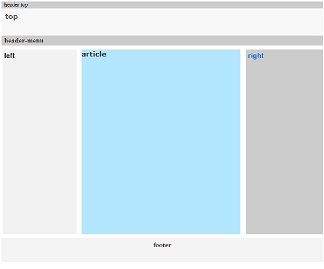
Find here examples of creative and unique website layouts.
Free CSS HTML Menu
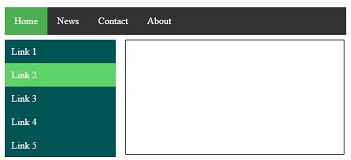
Find here examples of creative and unique website CSS HTML menu.